
sort performance
what is the performance of sort function of this toolbox?
Are there any comparison studies?

We use quicksort algorithm, which is pretty much de-facto standard in all programming languages.
If we compare sort speed of our toolbox and VPA:
>> A = vpa(rand(10000,1));
>> tic; sort(A); toc;
Elapsed time is 0.065205 seconds.
>> A = rand(10000,1,'mp');
>> tic; sort(A); toc;
Elapsed time is 0.003493 seconds.
So, our toolbox is ~19 times faster.
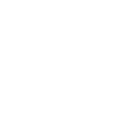
arrayfun
is there a way to use arrayfun for mp? I need to define a function in integral form. but integral only uses scalar values in its limits. If I use loops it would be a little slower.
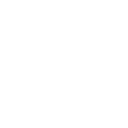
eigs() bug
I noticed a pretty severe bug for the eigs() function. In particular, if I do:
A = sparse(mp(eye(4),34)); [evecs,evals] = eigs(A,1);
I expect an identity matrix to be returned for both evecs and evals, however, I get the following result after one call of the above code:
evecs = 0.6575264478049146996368762588811955 0.1350828341320901021606099191810989 0.09164029388649532440547542667314778 0.7355363042680420857014721043910981 evals = 1
If I call it again I get an error:
Error using mp/subsref (line 1375) Index exceeds matrix dimensions. Error in mpeigs (line 523) D = ordeig(H(r,r)); Error in mp/eigs (line 3763) [varargout{1:nargout}] = mpeigs(varargin{:});
and in general it seems very sporadic, random, and most importantly, incorrect each time I call it. However, if I instead use the same function but without specifying the "1" as a parameter, e.g.:
A = sparse(mp(eye(4),34)); [evecs,evals] = eigs(A);
Everything seems to work as expected and I get identities returned for both evecs and evals. Obviously I don't need the eigenvalues and eigenvectors of the identity matrix but this is giving me little confidence in the eigenvectors and eigenvalues returned for the actual matrices I'm interested in. Am I missing something here or calling the function incorrectly? Also, I've noticed similar issues with the generalized eigenproblem equivalent of the above example.
Thank you.
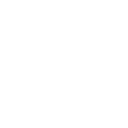
Saving leads to corrupt file
I have a loop in which I generate approximately 100 mp matrices with sizes ranging form N = 4 to ~1500. In order to use them later I save them as cell entries (A{i} = A). In some instances these will save, other times I get a corrupt file error warning.
It always happens with the same matrices, just wth some parameters I get the errors with others I don't.
Any ideas or suggestions?
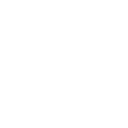
Compilation in standalone application
Wonder if it is possible, currently, to compile your libraries using MCC for eventual deployment as a standalone application.
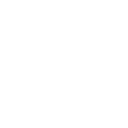
eigs() support for sigma = 'sa'
I've noticed that when using advanpix data structures with eigs(), I get erroneous results when requesting the smallest eigenvalue.
Please refer to the following code using Matlab's data structure:
A_matlab = speye(25,25); %initialize Matlab sparse identity matrix A_matlab(13,13) = 1/3; eigs(A_matlab,1,'sa') %request smallest eigenvalue
When running this code, I receive the following output:
ans = 0.333333333333333
Now consider a similar experiment with Advanpix:
A_advanpix = mp(speye(25,25),32); %initialize Advanpix sparse identity matrix A_advanpix(13,13) = mp('1/3'); eigs(A_advanpix,1,'sa') %request smallest eigenvalue
When running this code, I receive the following output:
ans = 0
I have noticed if I replace '1/3' with '-1/3' in the above experiments, I obtain the correct results, namely both return "-1/3" in the appropriate precision. I appreciate any help with this issue, thank you for your assistance!
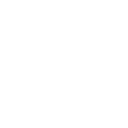
Slow vectorized operations compared to Matlab double
I've noticed when doing vectorized operations with advanpix mp data structures, it's extremely slow compared to Matlab. For example, consider the follow code where I initialize 1000x1000 sparse, random matrices using both Matlab double and advanpix double data structures:
A_matlab = sprand(1000,1000,.2);
A_advanpix = mp(sprand(1000,1000,.2),16);
I then time the operation of "zeroing out" the first 100 rows in each
tic
A_matlab(1:100,:) = 0;
toc Elapsed time is 0.008518 seconds.
tic
A_advanpix(1:100,:) = 0;
toc Elapsed time is 2.534518 seconds.
Of course, this naturally gets much worse as the size of A increases. Is this behavior expected or am I using the advanpix data structures incorrectly?
Thank you!
Joe
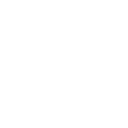
Specifying the number of bits instead of number of digits
Is it possible to specify the number of bits used to represent numbers, rather than the number of decimal digits?

Not directly, but you can convert required number of bits to decimal digits using simple formula:
>> d = floor(bits*log10(2)); % precision in decimal digits >> mp.Digits(d)
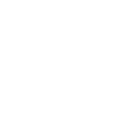
How is a double precision number extended?
>> mp(1/3) % Accuracy is limited to double precision ans = % since 1/3 was evaluated in double precision first 0.33333333333333331482961625624739099293947219848633
How are the extended digits, i.e. 1482961625624739099293947219848633, created? Are they totally random numbers or some of them are determined by the guard digits in the double-precision representation of 1/3?
I've been thinking that a natural extension would be done by trailing zeros, resulting, for example, in the example above, something like:
0.333333333333333300000000000000000000000000000000
Any discussion/comment is welcome. Thanks!

Toolbox uses binary representation of floating-point numbers.
When we extend double precision number with zeroes (in binary representation) - it doesn't refer to zeros in decimal representation (please refer to some guides on floating-point numbers & their formats for deeper understanding).
Do not use mp(1/3) because 1/3 is computed in double precision and then converted to quadruple - final value has double precision accuracy (not the intended quadruple).
The correct way to compute constants to full accuracy is:
>> mp.Digits(34); >> mp('1/3') ans = 0.3333333333333333333333333333333333 >> mp.Digits(50); >> mp('1/3') ans = 0.33333333333333333333333333333333333333333333333333 >> mp.Digits(70); >> mp('1/3') ans = 0.3333333333333333333333333333333333333333333333333333333333333333333333
In this case, double precision constant is not extended by zeros (in binary format) but computed with full accuracy from the onset (within limits of specified precision).
***
Could you please reply to another thread you started yesterday: https://mct.userecho.com/communities/1/topics/179-it-would-be-fantastic-if-quadeig-is-supported
We prioritize only well-motivated requests to add new functionality.

R2019b linux problem
On R201b (Linux Mint 19.2) is the following problem:
>> mp.Test
mp.Digits() : <- success
mp.GuardDigits() : <- success
double() : <- success
int8() : <- success
uint8() : <- success
int16() : <- success
uint16() : <- success
int32() : <- success
uint32() : <- success
int64() : <- success
uint64() : <- success
colon() : <- success
plus() : <- success
minus() : <- success
times() : <- success
mtimes() : <- success
rdivide() : <- success
ldivide() : <- success
mldivide() : <- success
mrdivide() : <- success
mpower() : <- success
power() : <- success
realpow() : <- success
transpose() : <- success
ctranspose() : <- success
uminus() : <- success
uplus() : <- success
sin() : <- success
cos() : <- success
tan() : <- success
sec() : <- success
csc() : <- success
cot() : <- success
acos() : <- success
asin() : <- success
atan() : <- success
acot() : <- success
atan2() : <- success
hypot() : <- success
cosh() : <- success
sinh() : <- success
tanh() : <- success
sech() : <- success
csch() : <- success
coth() : <- success
acosh() : <- success
asinh() : <- success
atanh() : <- success
acoth() : <- success
asech() : <- success
acsch() : <- success
exp() : <- success
expm1() : <- success
log() : <- success
log10() : <- success
log1p() : <- success
log2() : <- success
nextpow2() : <- success
pow2() : <- success
sqrt() : <- success
reallog() : <- success
realsqrt() : <- success
nthroot() : <- success
pow2(F,E) : <- success
min(), max() : <- success
prod(matrix) : <- success
prod(vector) : <- success
sum(matrix) : <- success
sum(vector) : <- success
cumsum(matrix) : <- success
cumsum(vector) : <- success
cumprod(matrix) : <- success
cumprod(vector) : <- success
dot() : <- success
cross() : 2 <- fail
3 <- fail
9 <- fail
10 <- fail
<- success
svd() : <- success
qr() : <- success
lu(square) : <- success
lu(rect) : <- success
pinv() : <- success
null() : <- success
balance() : <- success
eig() : <- success
qz() : <- success
hess() : <- success
chol() : <- success
schur() : <- success
ordschur() : <- success
rank() : <- success
trace() : <- success
det() : <- success
inv() : <- success
sort(real) : <- success
sort(complex) : <- success
find() : <- success
<,<=,>,>=,==,~= : <- success
and,or,not,xor : <- success
all() : <- success
any() : <- success
isinf() : <- success
isnan() : <- success
isfinite() : <- success
isreal() : <- success
abs() : <- success
sign() : <- success
conj() : <- success
angle() : <- success
imag() : <- success
real() : <- success
complex() : <- success
ceil() : <- success
fix() : <- success
floor() : <- success
idivide() : <- success
round() : <- success
rem() : <- success
mod() : <- success
tril(matrix) : <- success
tril(vector) : <- success
triu(matrix) : <- success
triu(vector) : <- success
diag(matrix) : <- success
diag(vector) : <- success
norm(matrix) : <- success
norm(vector) : <- success
cond() : <- success
rcond() : <- success
factorial() : <- success
mean() : <- success
std() : <- success
erf() : <- success
erfc() : <- success
erfi() : <- success
FresnelS() : <- success
FresnelC() : <- success
gammaln() : <- success
gamma() : <- success
gammainc() : <- success
psi() : <- success
zeta() : <- success
eint() : <- success
logint() : <- success
cosint() : <- success
sinint() : <- success
besselj() : <- success
bessely() : <- success
besseli() : <- success
besselk() : <- success
besselh() : <- success
hypergeom() : <- success
KummerM() : <- success
KummerU() : <- success
expm() : <- success
logm() : <- success
sqrtm() : <- success
sinm() : <- success
cosm() : <- success
sinhm() : <- success
coshm() : <- success
fft : <- success
ifft : <- success
fft2 : <- success
ifft2 : <- success
Customer support service by UserEcho